The Big O Notation

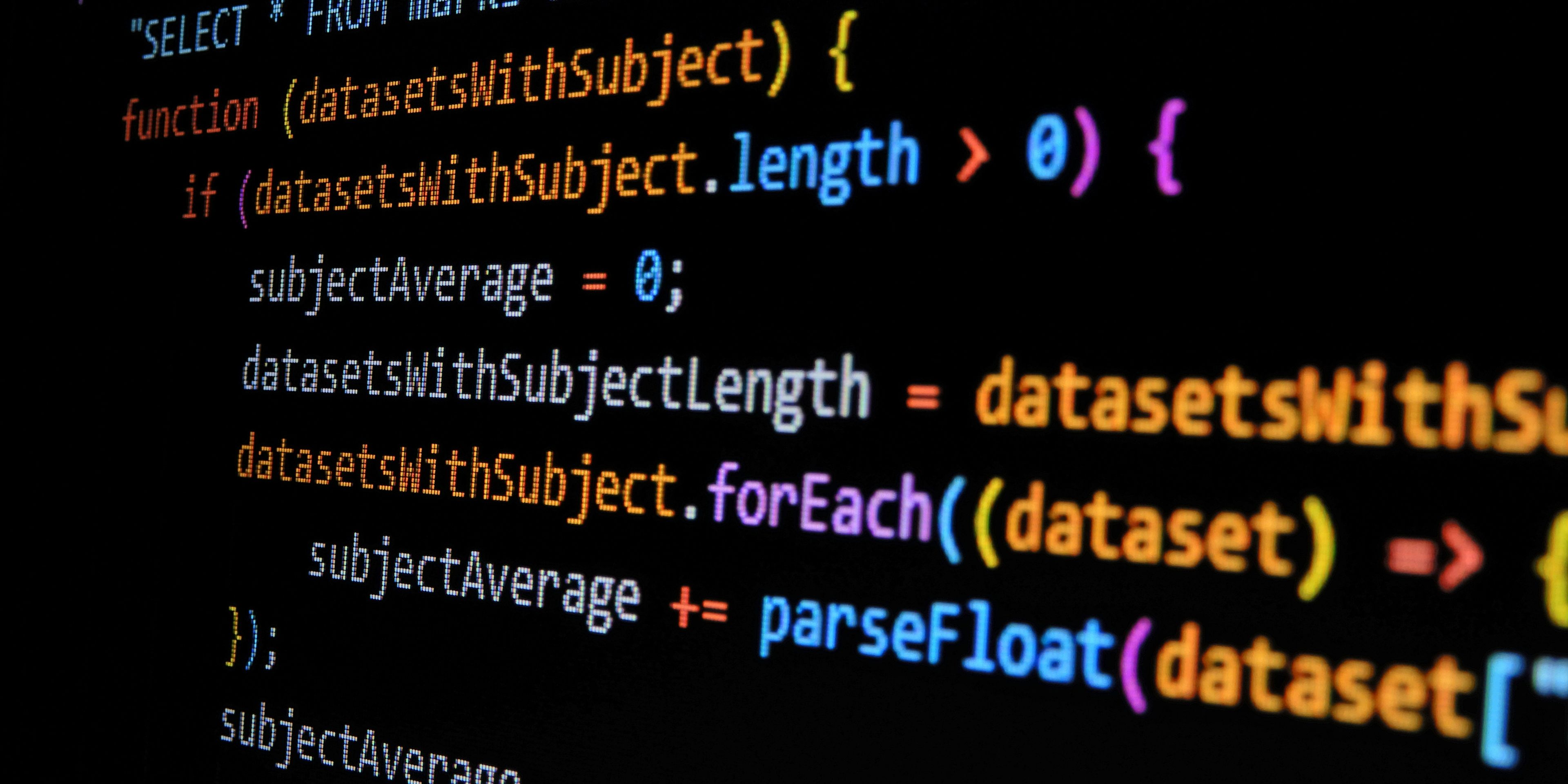

Hello, fellow coders! Today, we're diving into a topic that sounds a bit intimidating at first but is incredibly useful for optimizing our code: Big O notation. Whether you're prepping for job interviews or just looking to understand your code's performance better, mastering Big O notation is essential. Let's break it down into friendly, bite-sized pieces and explore some practical JavaScript examples to make it all clear.
What is Big O Notation?
Big O notation is a mathematical concept used in computer science to describe how well an algorithm scales as the amount of data processed increases. In simpler terms, it tells you how the runtime or space requirements of your program grow as the input size grows. It’s like predicting traffic on your route home; wouldn't it be nice to know what to expect as rush hour approaches?
Common Big O Types
- O(1) – Constant Time: No matter how much data, the operation takes the same time.
- O(n) – Linear Time: The time increases linearly with the amount of data.
- O(log n) – Logarithmic Time: Increases logarithmically; great for large data sets.
- O(n^2) – Quadratic Time: Time increases quadratically; watch out, it can slow down quickly with large data!
Let’s See Some Code!
To make this more concrete, let's look at some common scenarios in JavaScript:
Example 1: Constant Time (O(1))
function getFirstItem(array) {
return array[0]; // Accessing the first element is always constant time
}
No matter the size of the array, fetching the first element always takes the same amount of time.
Example 2: Linear Time (O(n))
function findItem(array, item) {
for (let i = 0; i < array.length; i++) {
if (array[i] === item) {
return i; // The time to find the item scales linearly with the array's size
}
}
return -1; // Item not found
}
As the array grows, the worst-case scenario (item is at the end or not present) means looking through every element.
Example 3: Logarithmic Time (O(log n))
function binarySearch(sortedArray, item) {
let low = 0;
let high = sortedArray.length - 1;
while (low <= high) {
let mid = Math.floor((low + high) / 2);
let guess = sortedArray[mid];
if (guess === item) {
return mid;
}
if (guess > item) {
high = mid - 1;
} else {
low = mid + 1;
}
}
return -1; // Item not found
}
Binary search is a classic example of O(log n). It repeatedly divides the search interval in half until it finds the target.
Example 4: Quadratic Time (O(n^2))
function bubbleSort(array) {
let n = array.length;
for (let i = 0; i < n; i++) {
for (let j = 0; j < n - i - 1; j++) {
if (array[j] > array[j + 1]) {
let temp = array[j];
array[j] = array[j + 1];
array[j + 1] = temp;
}
}
}
return array;
}
Bubble sort compares each pair of adjacent items and swaps them if they are in the wrong order, which means its runtime grows quadratically with the size of the input.
Wrap-Up
Big O notation helps us understand the performance implications of our algorithms, which becomes crucial as our applications scale. While some algorithms might seem fast on small inputs, Big O reveals their true colors as those inputs grow. By keeping these concepts in mind, you can write more efficient and scalable code—something that both your application users and your future self will thank you for!
Happy coding!