Maximum Subarray Problem - O(n^2) vs O(n)

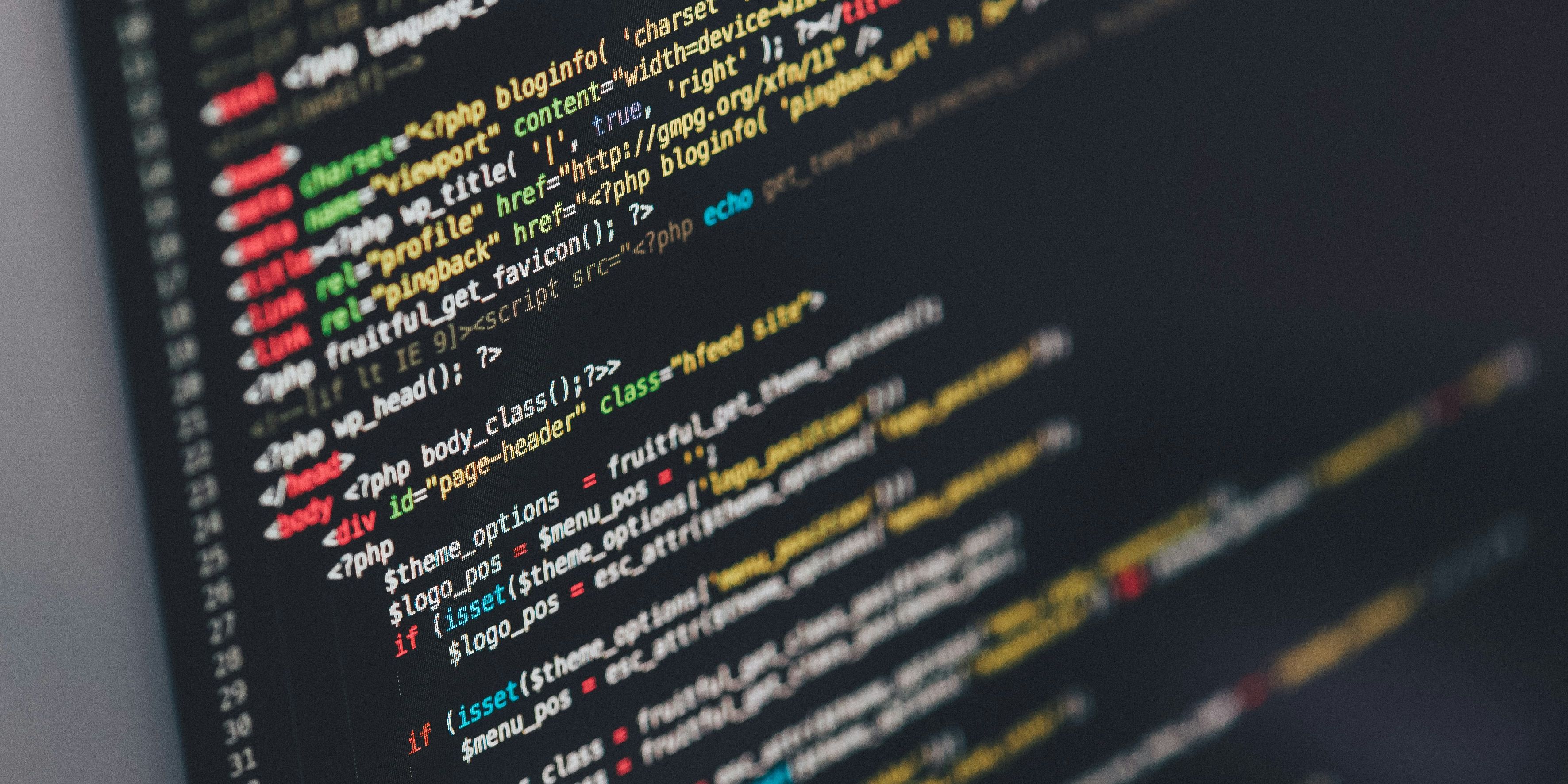

In the realm of computer science, the Maximum Subarray Problem is a classic. It's a problem that has been tackled by many, and it's a great way to understand the power of different algorithmic approaches. In this post, we'll delve into two solutions: the O(n^2) and the O(n) approaches, and see how they stack up against each other.
The Problem
The Maximum Subarray Problem is quite straightforward. Given an array of integers, the task is to find the contiguous subarray with the largest sum. For example, for the array [-2, 1, -3, 4, -1, 2, 1, -5, 4], the contiguous subarray with the largest sum is [4, -1, 2, 1], with a sum of 6.
The O(n^2) Approach
The O(n^2) approach is the brute force method. It involves generating all possible subarrays, calculating their sums, and then returning the maximum sum. While this method is simple and straightforward, it's not very efficient, especially for large arrays.
bruteForce.js
function maxSubArray(nums) {
let maxSum = -Infinity;
for (let i = 0; i < nums.length; i++) {
let sum = 0;
for (let j = i; j < nums.length; j++) {
sum += nums[j];
maxSum = Math.max(maxSum, sum);
}
}
return maxSum;
}
The O(n) Approach
The O(n) approach, also known as Kadane's algorithm, is a more efficient solution. It involves iterating through the array once, keeping track of the maximum sum and the current sum at each step. If the current sum becomes negative, it is reset to zero.
kadanesAlgorithm.js
function maxSubArray(nums) {
let maxSum = nums[0];
let currentSum = nums[0];
for (let i = 1; i < nums.length; i++) {
currentSum = Math.max(nums[i], currentSum + nums[i]);
maxSum = Math.max(maxSum, currentSum);
}
return maxSum;
}
In conclusion, while both approaches solve the problem, the O(n) approach is more efficient and scales better with larger arrays. It's a great example of how a clever algorithm can drastically improve performance. So, next time you're faced with a problem, remember to consider different approaches - you might find a more efficient solution just around the corner!
What are your thoughts on these two approaches? Have you encountered the Maximum Subarray Problem before? How did you solve it? Share your experiences and insights, and let's learn together!